Guide: Setting up a Rails API Backend
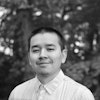
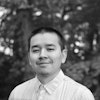
While this guide will walk a reader through creating a Rails API, it does assume the reader has their computer set up for a programming environment. This is not a guide on how to install things like Ruby, Rails, or Postgresql.
To begin creating any Rails application, we need to enter a few commands in the terminal. This command tells the computer to make a new directory (mkdir) called list-app (this is going to be the name of my application, so this can be changed to whatever you desire. This command tells the terminal to change to the newly created directory so that future commands will be run in the correct location. This is a Rails command that creates the new application in the current directory. The additional options included at the end of the command will create an API version of the Rails application and also assign the database to be postgresql instead of the default mysql. The change in database is important because it makes a future step of deploying to Heroku easier.
mkdir list-app
cd list-app
rails new list-app --api --database=postgresql
Once Rails is finished files and installing its dependency gems, which could take a few minutes, we need to tell the system to create a local database with the command
rake db:create
Now go ahead and start up the local server
rails server -p 3001
We are running the Rails server on port 3001 instead of the default port to avoid any conflicts down the road if/when React components get added.
Schema
With the Rails API setup, we now need to add some migrations to our application to create our database structure. Let’s start with the migration for our User table. Now we need to add the columns that will be in our users table; I’ll be adding a username, email and password column to my table. This causes the file to look like
rails g migration CreateUsers
The password_digest column is a requirement of our Bcrypt gem, otherwise a simple password column would work. The timestamps will create two columns, one that stores the date and time when a new record is created and one that stores the date and time when a record is edited. Next we need to create a migration for our list table. This table will have a title, a status, and will belong to a user. Lastly we need to create a migration for our list items table. This table will have a name, quantity, status and will belong to a list. Our migration file should look like
With the migration files ready to go, there is one last thing to do before moving on to the next step. Running the following command will instruct Rails to run the migration files and create the tables and columns in the database.
rake db:migrate
The next step of creating a Rails API is setting up the models, we will be starting with the User model. Navigate to the model folder, it is nested under the app folder in the root of the directory. In the model folder we need to create a user.rb
file to house our User model. In order to have Rails work its magic, each model file will need to contain a class of the same name.
Controllers
With all the models setup, we are going to move to crafting our controllers so we have CRUD capabilities. We need to create a controller for each of our models, except the User model which is handled slightly differently; these files need to be located in the controller folder that is nested in the app folder in order for Rails magic to be successful. The User model will have two controllers, one to handle users logging in and out, Sessions Controller, and one to handle user registration and account deletion, Registrations Controller. The Sessions and Registrations Controllers will be kept simple for this example because the end result app will only allow users to sign up, log in and log out. We need to have our controllers render json instead of HTML since our Rails backend is in API mode.
The ApplicationController needs to be modified slightly. Since we are using session to store user_id upon login, we need to add a way to access the current user in all of our controllers. With all of our controllers inheriting from the ApplicationController, it is the perfect location to house this method. With our methods to check for the current user, we can not setup our Lists Controller. We want users to be able to see all of their lists, view a specific list, create new lists, update lists and also delete lists. We also don’t want users to be able to do any of those things unless the list belongs to them, so we need to check the current user id vs the user id provided in params before performing an action. This results in the Lists Controller looking like
Routes
With the migrations, models, controllers, and serializers setup, the final step is to construct the routes so our backend knows how to direct traffic and what information to provide when queried. Convention is to have the routes for APIs to be nested under ‘/api’
We do not want to create routes which lead to dead ends so we need to make sure to restrict which routes are available for each model and there needs to be routes for login, logout and signup. With these guidelines in mind, here is what the routes.rb file should look like
If you’ve followed the article to this point then take a quick moment for some celebration because there was a lot of material covered in this article. Thanks for reading!